I am working on a password manager for managing personal passwords using just one master password.
Using a password manager increases security and reduces the risk of password theft.
The program utilizes the Web Crypto API to encrypt and decrypt passwords with AES-GCM.
Passwords are extracted from a dBase file (DBF).
The encrypted passwords are encrypted using an encryption key and an initialization vector (IV) as parameters.
In the DBF file, the encrypted password and the initialization vector are stored.
Both values are retrieved from the DBF file using an AJAX request. Decryption takes place on the client-side in the web browser.
The encryption key is not stored; it is the master password and must always be entered by the user.
However, it is essential to choose a secure master password for the password manager itself, as it grants access to all stored passwords.
Passwords can be categorized based on the contexts in which they may be used.
Here are some examples of password categories that you could use for personal, social, and business purposes:
Personal Passwords:
1. Personal email address
2. Social media (e.g., Facebook, Twitter, Instagram)
3. Online shopping (e.g., Amazon, eBay)
4. Entertainment sites (e.g., Netflix, YouTube)
5. Personal Wi-Fi network
Social Passwords:
1. Forums and discussion groups (e.g., Reddit, Stack Overflow)
2. Online games (e.g., Steam, PlayStation Network)
3. Chat apps (e.g., WhatsApp, Discord)
Business Passwords:
1. Business email address
2. Company network
3. Business software or tools
4. Work-related cloud services (e.g., Google Workspace, Microsoft 365)
5. Business social media or websites
Other categories could include:
1. Banking and financial accounts
2. Healthcare and medical services
3. Educational institutions (e.g., university accounts)
4. Government or agency access
Best regards,
Otto
Code: Select all | Expand
$(document).ready(function() {
passwordBtn.on('click', function() {
var putData = {};
putData["request"] = "edit";
var row = getSelections()[0];
var id = row.id;
var cIdx = id.toString();
putData["id"] = cIdx;
console.log("putData:", putData); // Konsolenausgabe zum Testen
$.ajax({
url: "./getRecord.prg",
type: "PUT",
dataType: "json",
data: JSON.stringify(putData),
success: function(data) {
console.log("Response:", data);
var obj = data.array[0];
encryptedPassword = obj.link;
if (encryptedPassword !== null) {
console.log('Verschlüsseltes Passwort:aus dem DBF file', encryptedPassword);
// const iv = "8fbc9ec521b300fe9d845987";
// Convert the hexadecimal string 'iv' to a Uint8Array
const ivHexString = "8fbc9ec521b300fe9d845987";
const iv = new Uint8Array(ivHexString.match(/.{1,2}/g).map(byte => parseInt(byte, 16)));
const encryptionKey = new TextEncoder().encode('dummy_key_123456'); // Dummy-Verschlüsselungsschlüssel!
// Passwort entschlüsseln
decryptPassword(encryptedPassword, encryptionKey, iv)
.then(decryptedPassword => {
if (decryptedPassword !== null) {
console.log('Entschlüsseltes Passwort:', decryptedPassword);
alert( decryptedPassword );
} else {
console.log('Fehler bei der Entschlüsselung.');
}
});
} else {
console.log('Fehler bei der Verschlüsselung.');
}
},
error: function(jqXHR, textStatus, errorThrown) {
console.error("AJAX-Fehler:", textStatus, errorThrown);
alert("Fehler beim Lesen");
}
});
});
});
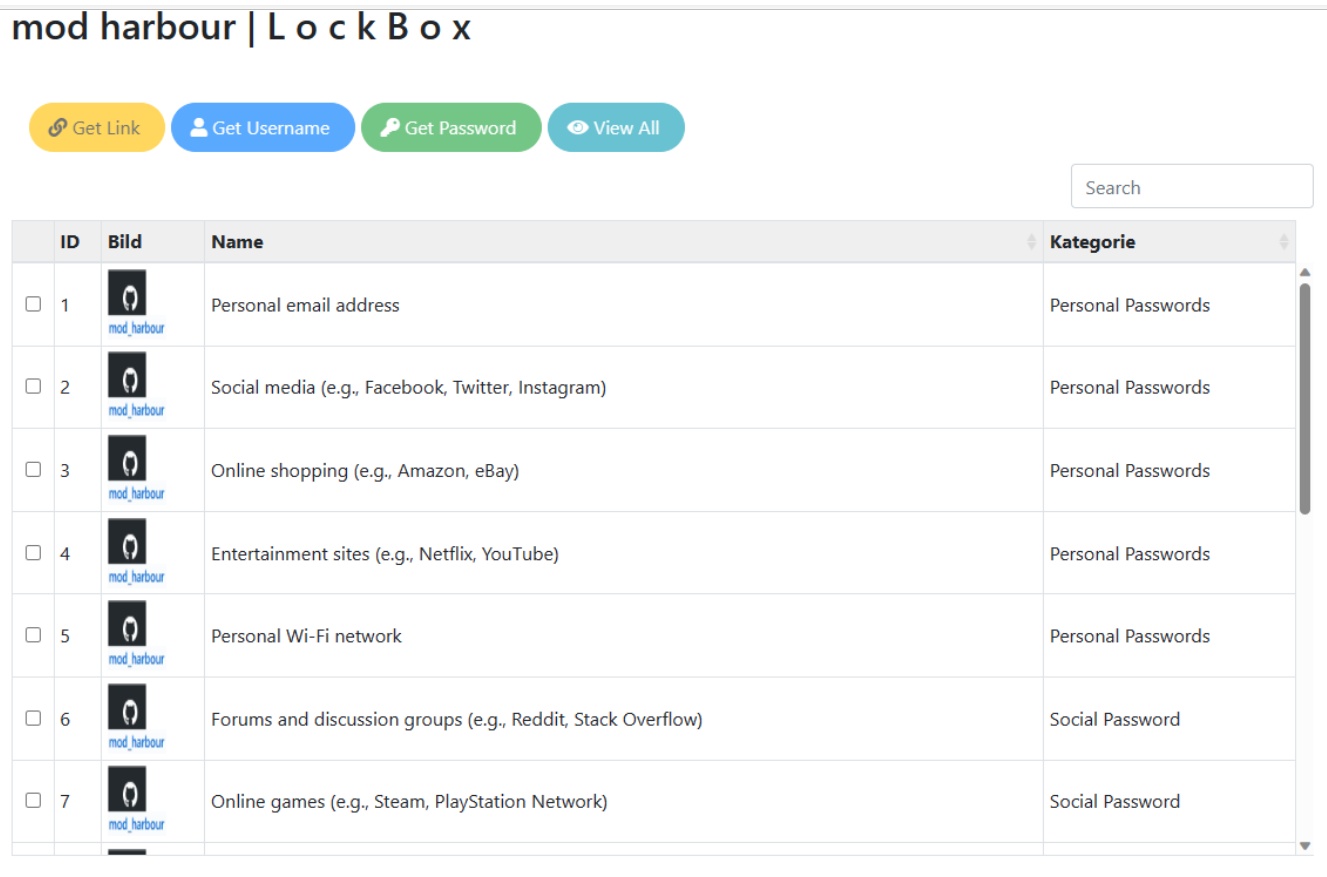